Python Server Side Programming Programming NameErrors are raised when your code refers to a name that does not exist in the current scope For example, an unqualified variable name The given code is rewritten as follows to catch the exception and find its typeErrors in Python can be categorized into two types 1 Compile time errors – errors that occur when you ask Python to run the application Before the program can be run, the source code must be compiled into the machine codeLastly I hope this tutorial on Python logging was helpful So, let me know your suggestions and feedback using the comment section References I have used below external references for this tutorial guide docspythonorg Logging Configuration File docspythonorg Configure Logging docspythonorg Basic Python Logging Tutorial Mastering Python
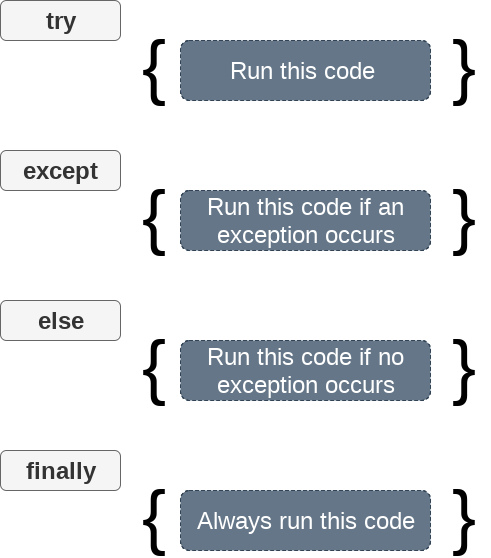
Python Exception Handling Python Try Except Javatpoint
Name error python example
Name error python example-And here is our program At each iteration of the while loop we Calculate the nth term as the sum of the (n2)th and (n1)th terms Assign the value of the (n1)th terms to the (n2)th termsAn example of an runtime error is the division by zero Consider the following example x = float (input ('Enter a number ')) y = float (input ('Enter a number ')) z = x/y print (x,'divided by',y,'equals ',z) The program above runs fine until the user enters 0 as the second number
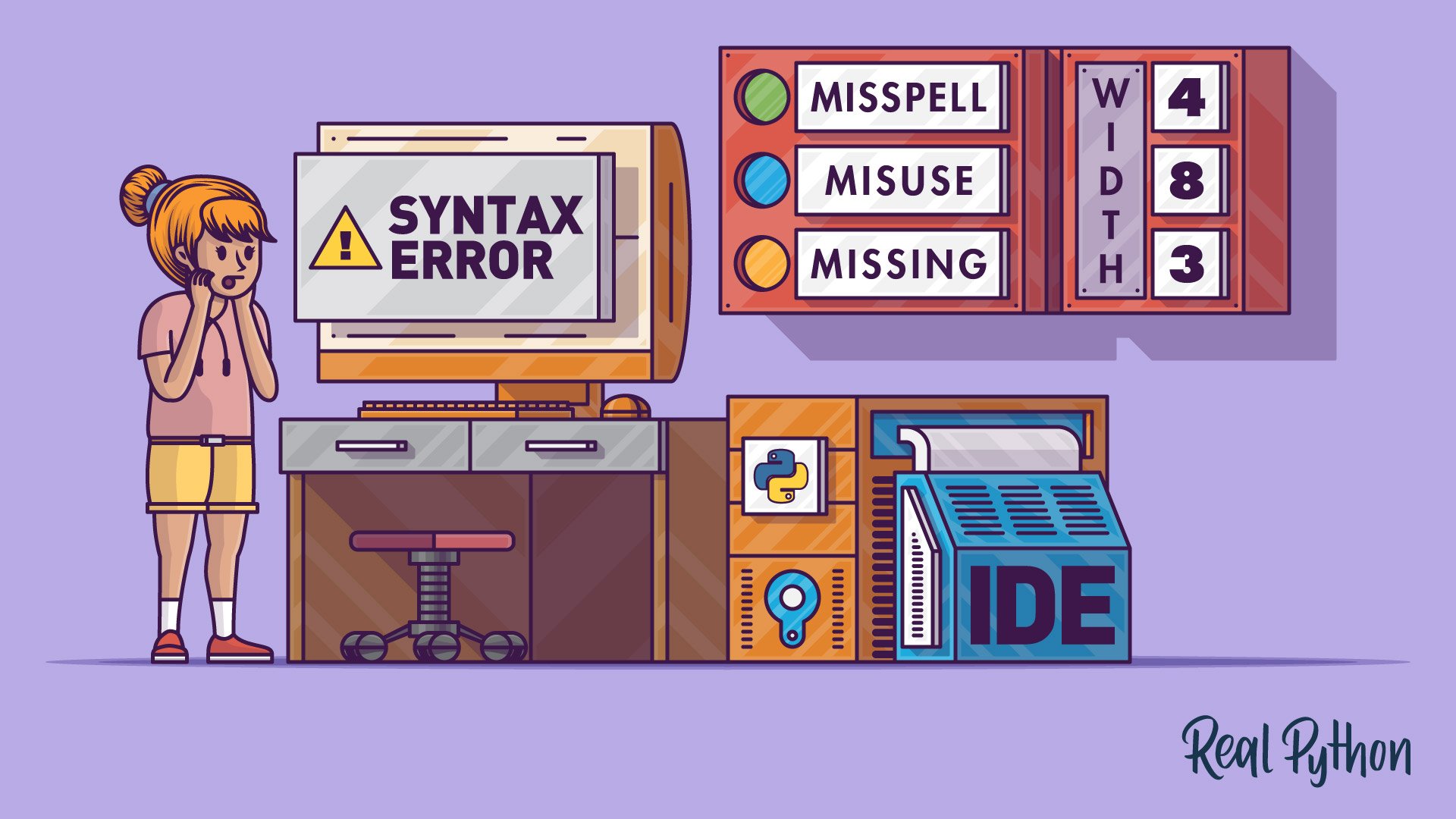


Invalid Syntax In Python Common Reasons For Syntaxerror Real Python
When the Python interpreter reads a file, the __name__ variable is set as __main__ if the module being run, or as the module's name if it is imported Reading the file executes all top level code, but not functions and classes (since they will only get imported)In the output graphic, you can see program displayed salaries for emp ID 1 and 3 As I entered 5, it did not raise any exception (KeyError) Instead get method displayed the default messagePython NameError When you run Python code, you may get a NameError such as the following NameError name 'x' is not defined The x in the error will vary depending on your program The error means that Python looks for something named x but finds nothing defined by that name Common causes Common causes include you misspelled a variable name
# define Python userdefined exceptions class Error(Exception) """Base class for other exceptions""" pass class ValueTooSmallError(Error) """Raised when the input value is too small""" pass class ValueTooLargeError(Error) """Raised when the input value is too large""" pass # you need to guess this number number = 10 # user guesses a number until he/she gets it right while True try i_num = int(input("Enter a number ")) if i_num < number raise ValueTooSmallError elif i_num > numberHere's a list of common errors that result in runtime error messages which will crash your program 1) Forgetting to put a at the end of an if, elif, else, for, while, class, or def statement (Causes "SyntaxError invalid syntax") This error happens with code like this if spam == 42 print('Hello!') 2) Using = instead of ==Explanation In the above program, we are printing the current time using the time module, when we are printing cure time in the program we are printing current time using timelocal time() function which results in the output with year, month, day, minutes, seconds and then we are trying to print the value by changing the hours to a larger value the limit it can store
Traceback (most recent call last) File "example1py", line 2, in s = string(n) NameError name 'string' is not defined What we tried here is to convert a number to string But we all know that str (number) converts number to string but not string (number) This is kind of a typo error from the programmer point of viewName Error is raised when a local or global name is not found In the below example, ans variable is not defined Hence, you will get a name error try print (ans) except NameError print ("NameError name 'ans' is not defined") else print ("Success, no error!") NameError name 'ans' is not defined Runtime Error Not Implemented Error This section of the tutorial is derived from this Source Runtime Error acts as a base class for the NotImplemented ErrorIn this Python tutorial, we will discuss how to handle nameerror name is not defined in Python We will check how to fix the error name is not defined python 3 NameError name is not defined In python, nameerror name is not defined is raised when we try to use the variable or function name which is not valid Example value = 'Mango', 'Apple', 'Orange' print(values) After



Programmers Sample Guide Google App Engine Nameerror Global Name Execfile Is Not Defined
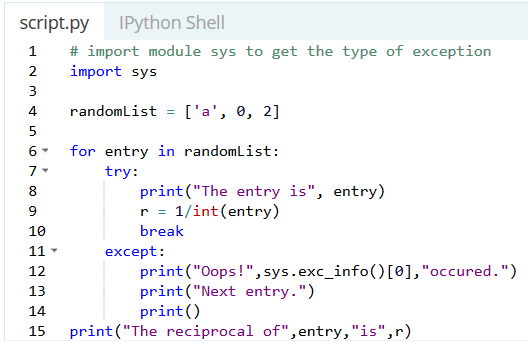


How To Perform Exception Handling In Python Packt Hub
Strengthen your foundations with the Python Programming Foundation Course and learn the basics To begin with, your interview preparations Enhance your Data Structures concepts with the Python DS CourseTutorialsTeachercom is optimized for learning web technologies step by step Examples might bePython Errors and Builtin Exceptions, Raised when the user hits the interrupt key ( CtrlC or Delete ) Python Standard Exceptions Here is a list all the standard Exceptions available in Python − Raised when the builtin function for a data type has the valid
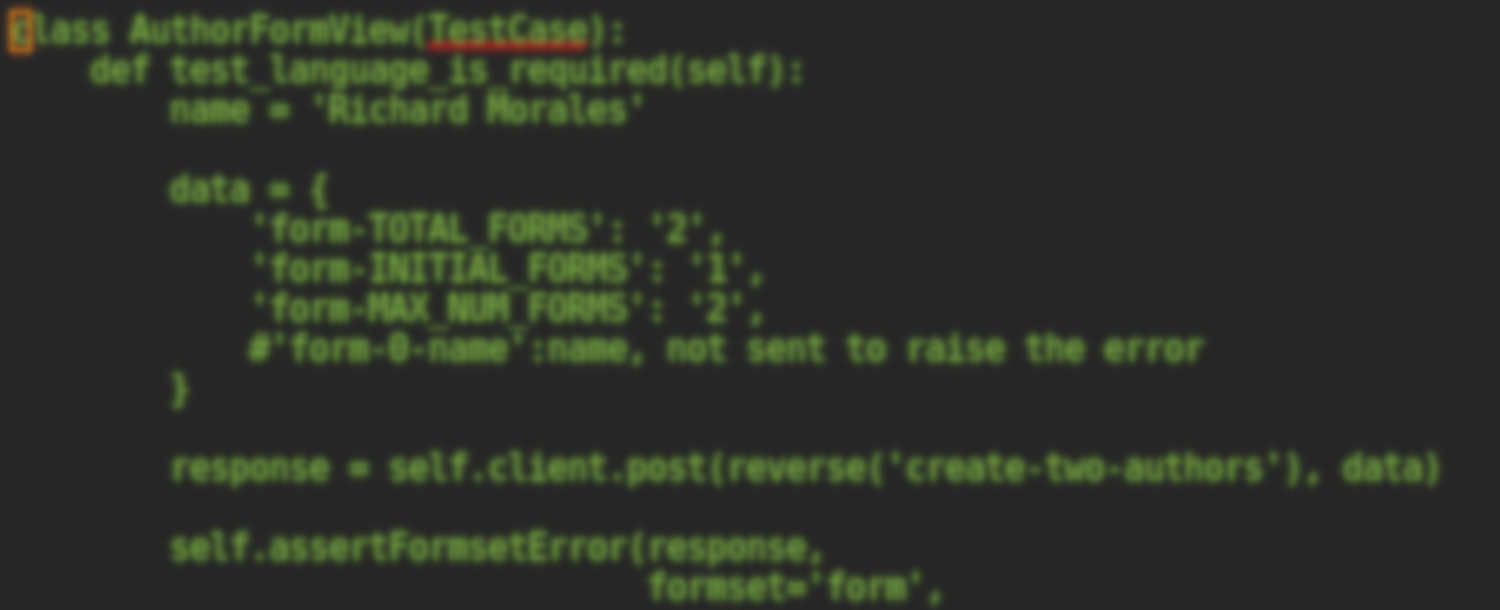


Django Formset Handling With Class Based Views Custom Errors And Validation Simple It Rocks



Python Database Exception Handling
2 The path of the module is incorrect 3 The Library is not installed 1 The name of the module is incorrect The first reason of this error is the name of the module is incorrect, so you have to check out the module name that you had imported For example, let's try to import Os module with double s and see what will happen >>> import oss Traceback (most recent call last) File "", line 1, in ModuleNotFoundError No module named 'oss'Since the name is denoted the code has successfully run without throwing the exception Below we have deleted the name denoted we can see the exception message thrown Code name='Smith' try print("Hello" " " name) except NameError print("Name is not denoted") finally print("Have a nice day") del name try print("Hello " name) except NameErrorWhat is machine learning;
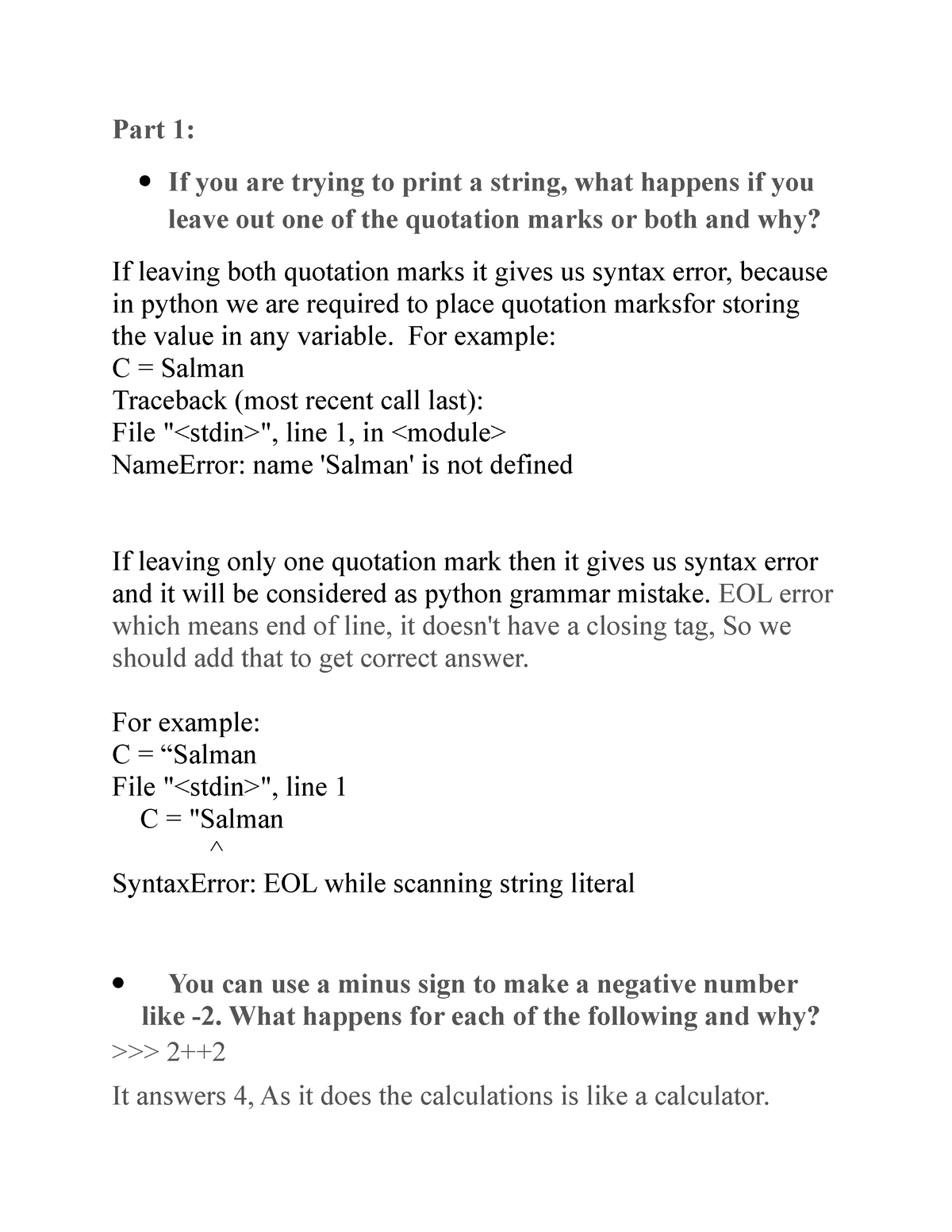


Python Learning Journal Unit 1 Part If You Are Trying To Print String What Studocu



How To Import Modules For Functions Held In A Separate Python Script Stack Overflow
Else work_loadappend (work_des) hours_workedappend (work_len) print "The work has been added to your work planning!" work_request = Work_plan (8, 2, "task1") Work_plan print work_load it comes up with the error NameError name 'work_load' is not defined python nameerror function ShareException handling in java;In the previous blog, we learned about Python Exception Handling using Try, Except, and Finally Statement This time we will be learning how we can make our custom Error/Exception in python



The Computing Zone 14 Debugging
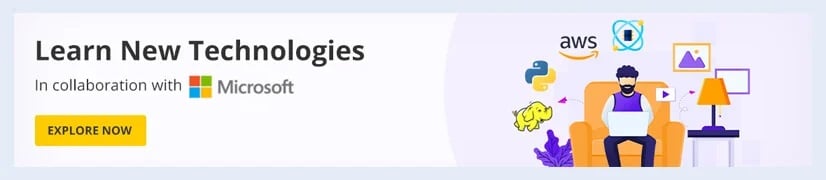


Variables In Python Global Static Variables Intellipaat
There are different kind of errors in Python, here are a few of them ValueError, TypeError, NameError, IOError, EOError, SyntaxError This output show a NameError >>> print 10 * ten Traceback (most recent call last) File "", line 1, in NameError name 'ten' is not defined and this output show it's a TypeError >>> print 1 'ten' Traceback (most recent call last) File "", line 1, in TypeError unsupported operand type (s) for 'int' and 'str'In the output graphic, you can see program displayed salaries for emp ID 1 and 3 As I entered 5, it did not raise any exception (KeyError) Instead get method displayed the default messageWell organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, PHP, Python, Bootstrap, Java and XML
.webp)


Typeerror Module Object Is Not Callable In Python
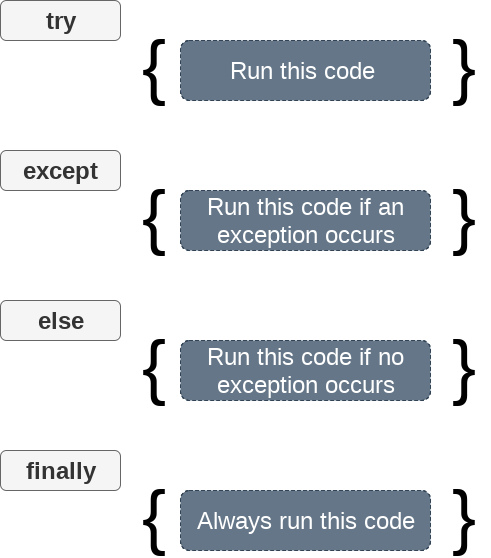


Python Exception Handling Python Try Except Javatpoint
0 件のコメント:
コメントを投稿